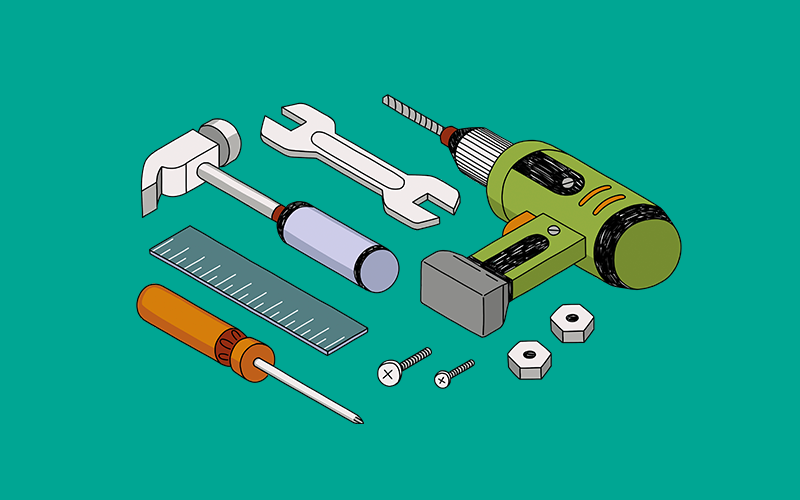
Adding UK Bank Account Validation to a WordPress Gravity Form
Unified Software’s BankVal UK plugin allows you to easily add a sort code and account number form to your site. However it can also be used to add our award winning validation to a preexisting form. This blog shows how to add validation to a Gravity form by adding a small amount of code to the functions.php file.
Once you have installed the plugin and entered your credentials you are ready to add our powerful account validation to your form (this blog uses a Gravity form but the principles should be the same for other types of form plug in). See our blog post on Integrating the bankvaluk WordPress plug-in form at https://www.unifiedsoftware.co.uk/blog/integrating-the-bankvaluk-wordpress-plug-in-form/ for more details on installing the plugin and entering your credentials.
As we will not be using the plugin’s own form there is no need to add a shortcode anywhere on your site. To integrate you just need to ‘plumb in’ to your existing workflow by using the gravity forms gform_validation hook and writing a short function for the functions.php file.
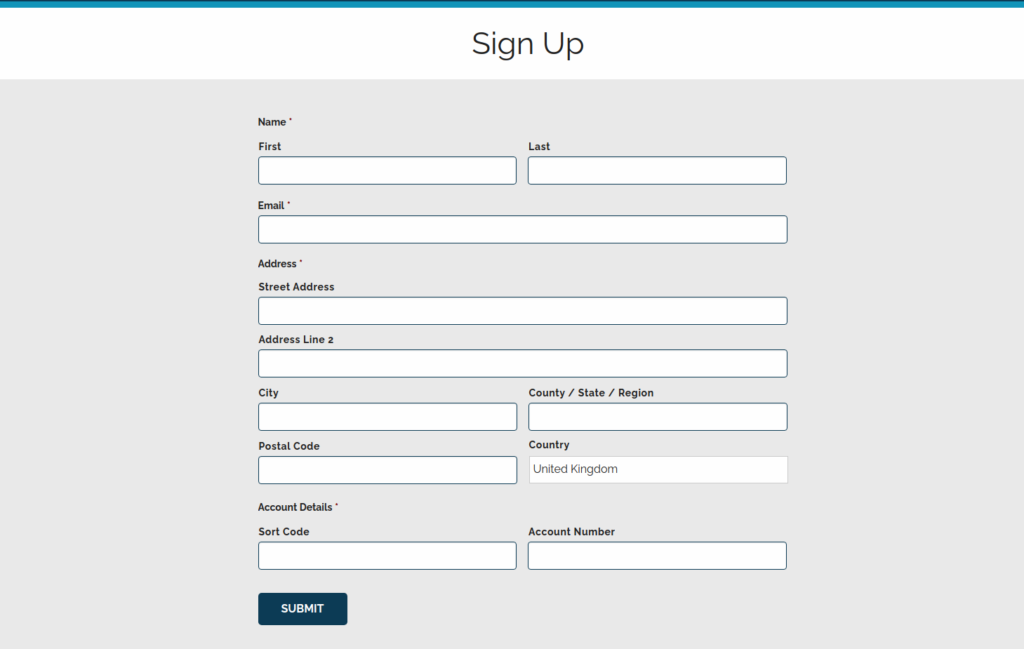
The Basics
For this example we have mocked up a form with contact information as well as the bank account details and some preexisting validation. To add BankVal validation to the form you will need three pieces of information from it:
The wordpress id of the form (in our case 6)
The id of the sortcode field (in our case its 8.3 but rgpost will need input_8_3)
The id of the account number field (in our case 8.6 or input_8_6)
Next add the following hook into the functions.php file (anywhere is fine, you may also want to create a child theme with its own functions.php file to avoid the function being overwritten if your main theme is updated):
add_filter('gform_validation_6','bank_val_uk');
The _6 on the end of the gform validation filter causes this filter to only be added to our Sign Up form. The string bank_val_uk is the name of our function we will add next, to tie the validation into the form. That function looks like this:
function bank_val_uk( $validation_result ) {
$form = $validation_result['form'];
$field = GFAPI::get_field($form, 8 );
$is_hidden = RGFormsModel::is_field_hidden($form, $field, array());
if ($is_hidden){
return $validation_result;
}
$sc = rgpost("input_8_3");
$ac = rgpost("input_8_6");
if(function_exists('UNIFIED_SOFTWARE\BANKVAL\validate_bank_account')){
$response = UNIFIED_SOFTWARE\BANKVAL\validate_bank_account($sc,$ac);
$is_valid=$response['result']==='VALID';
$is_verified=$response['result']==='VERIFIED';
$bankval=$is_valid||$is_verified;
} else {
error_log("can't access plugin");
}
if(!$bankval){
$validation_result['is_valid']=false;
$error_text = "Error";
switch($response['result']){
case "INVALID - Account":
$error_text = "Invalid Sortcode Account Number Combination!";
break;
case "INVALID - Sortcode":
$error_text = "Invalid Sortcode!";
break;
case "Error No Licence":
$error_text = "Validation not available";
break;
default:
$error_text = "Error Validating";
}
$field->failed_validation = true;
$field->validation_message = $error_text;
}
$validation_result['form'] = $form;
return $validation_result;
}
Ok, so that’s it, as long as you add in your form’s ids to the code above it will work. Our form will now validate bank account details at the point of capture. Thereby eradicating costly, time-consuming errors caused by mistyping or fraudulent behaviour.
But if you’re interested in whats going on above in more detail read on, we’ll take a look:
The Detail
function bank_val_uk( $validation_result ) {
$form = $validation_result['form'];
$field = GFAPI::get_field( $form, 8 );
This is our function signature. The $validation_result object is defined by the gform filters we invoked to call this function and is a PHP associative array. The parts of $validation_result we are interested in are form array and the is_valid flag. We also set a variable referencing the input field, in our case this field, 8 is a multi input field and references both the sort code and account. If you have single fields for each just set this to reference the sort code field.
$is_hidden = RGFormsModel::is_field_hidden( $form, $field, array() );
if ($is_hidden){
return $validation_result;
}
This section is optional, it is mainly in use to only perform the validation if the inputs are visible. For example if the form is in use outside of the uk or doesn’t always take uk bank account details. If the fields are hidden we simply return the $validation_result as it was passed in.
$current_page = rgpost( 'gform_source_page_number_' . $form['id'] ) ?
rgpost( 'gform_source_page_number_' . $form['id'] ) : 1;
$input_page = $field->pageNumber;
$is_hidden = RGFormsModel::is_field_hidden( $form, $field, array() )
|| $current_page != $input_page;
Optionally for multi-page forms it may also be necessary to include a check that the current page is the page the inputs are on. If this is your use case add the code above to the function. The first part grabs the current page being validated, the next gets the field’s page number, then finally we alter the is_hidden code to reuse the $field variable and incorporate the page check.
These 3 lines of code should replace the single $is_hidden line in the main function above. If you have a single page form where validation is always required you can skip all of the above apart from the lines setting the $form and $field variable and proceed as below.
$sc = rgpost("input_8_3");
$ac = rgpost("input_8_6");
Here we grab the values entered into the form using the ids of the inputs. In our case these are input_8_3 and input_8_6. Once we’ve grabbed these we need to check for the plugins availability.
if(function_exists('UNIFIED_SOFTWARE\BANKVAL\validate_bank_account')){
If it’s correctly installed we can go ahead and call bankval via the plugins validate_bank_account method.
$response = UNIFIED_SOFTWARE\BANKVAL\validate_account($sc,$ac);
$is_valid=$response['result']==='VALID';
$is_verified=$response['result']==='VERIFIED';
$bankval=$is_valid||$is_verified;
None of this code block is specific to gravity forms. So, if you have a different plugin or bespoke forms it still shows how to call the plugin validation.
The Response
The validate_bank_account method of the plugin takes the sort code and account number (it handles reading in your uname and pin from the plugin settings) and returns an array with a result field. That result field will be one of the following:
- VALID : A valid account number/sort code combination.
- INVALID – Sortcode : The sort code entered is not valid and does not exist in the EISCD.
- INVALID – Account : The sort code/account number combination is not valid.
- Error no licence : Your credentials are expired/incorrect.
- Error : Something else went wrong
Combining with the GForm validation
Once we have the response we save the valid/verified flags combined into a $bankval object then if that is false, i.e. the details aren’t valid we set the is_valid flag in the $validation_result array. The is_valid flag refers to the whole form, so it is important this is only set to false if this validation fails as just setting it to the value of $bankval would overwrite any previous validation, i.e. if the email address had failed its validation but the account was valid we would effectively remove the previous email validation.
In our example we alter the message based on the specific response message. However, you may, in the event of an error calling the service, not wish to stop the form from processing. In which case you should only set the is_valid flag inside the INVALID conditions
if(!$bankval){
$validation_result['is_valid']=false;
$error_text = "Error";
switch($response['result']){
case "INVALID - Account":
$error_text = "Invalid Sortcode Account Number Combination!";
break;
case "INVALID - Sortcode":
$error_text = "Invalid Sortcode!";
break;
case "Error No Licence":
$error_text = "Validation not available";
break;
default:
$error_text = "Error Validating";
}
To attach the error message to the correct part of the form we can reuse the $field variable we set earlier. In our case this is 8 and not 8_3 or 8_6 as we have used a multi input element. Once found we attach the error message and mark the field as invalid.
$field->failed_validation = true;
$field->validation_message = $error_text;
Finally we just need to set the form element to our changes and return it.
$validation_result['form'] = $form;
return $validation_result;
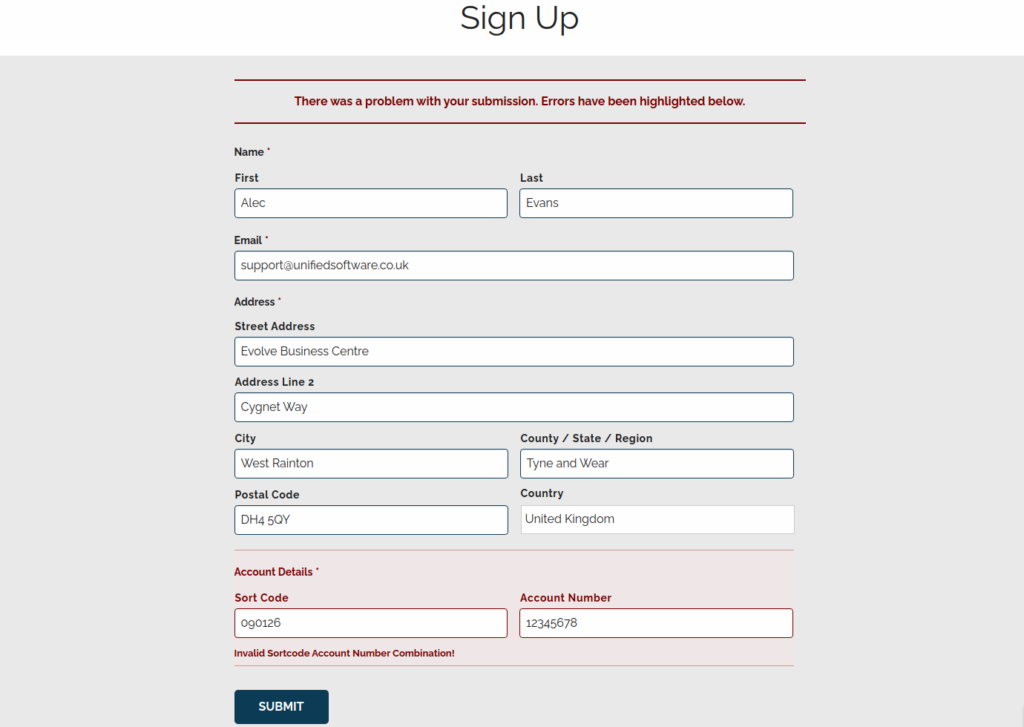
So that’s it, BankVal is integrated into our preexisting form. If you would like to see any other guides for specific WordPress forms, or plugins, to integrate BankVal or any of our other products please drop us a line. We can be reached at support@unifiedsoftware.co.uk.